USER MANAGMENT Component¶
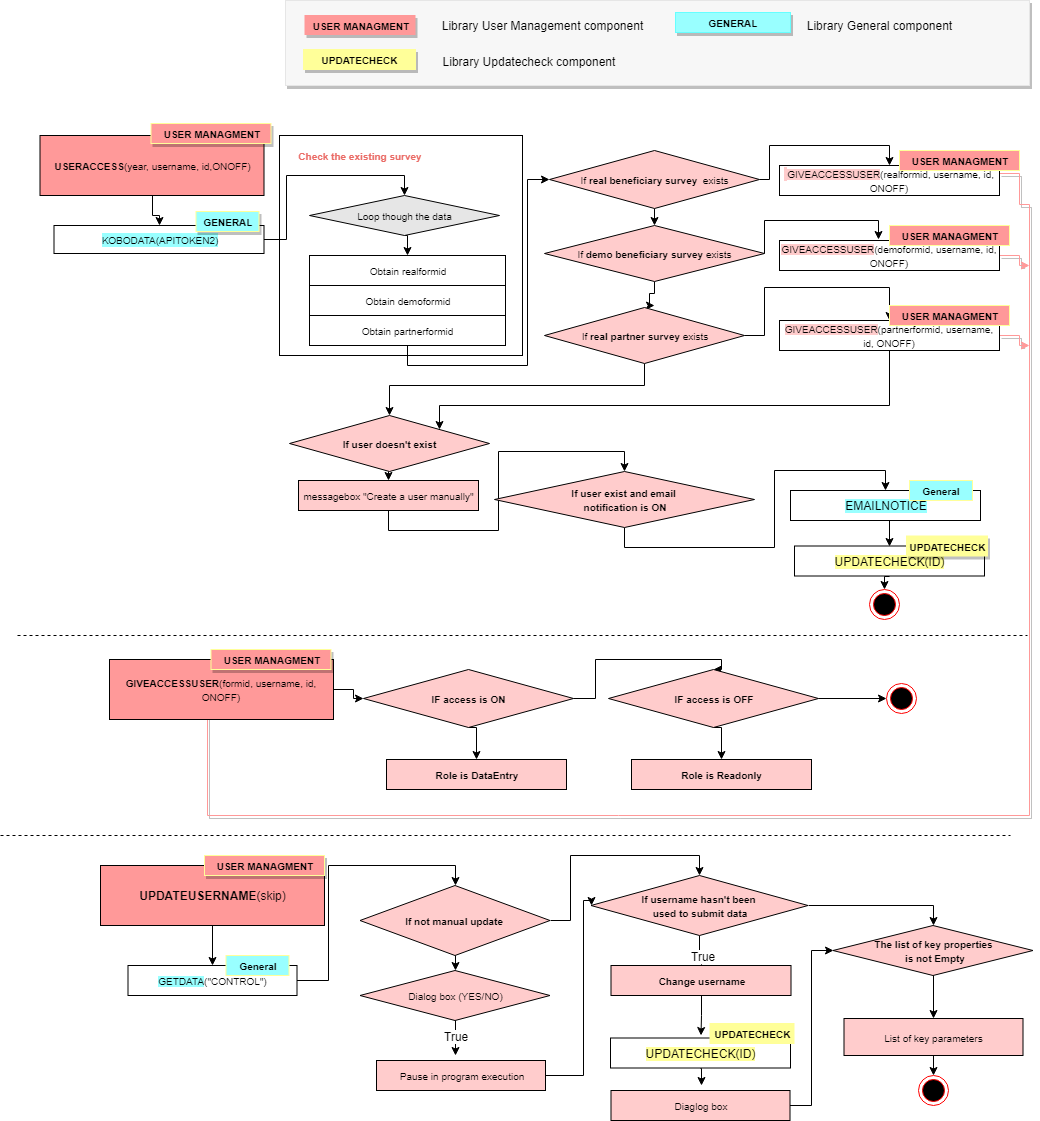
The block diagram of USER MANAGMENT Component¶
Warning
Update the function names
Function addUserAccess¶
-
addUserAccess
(year, username, id, ONOFF)¶ The function coordinates adding the users to completed template
- Arguments
year (string) – the current year
username (object) – the current username
id (type) – data id
ONOFF (type) – access with status ON/OFF (DataEntry/Readonly)
- Returns
string – result (User access updated”)
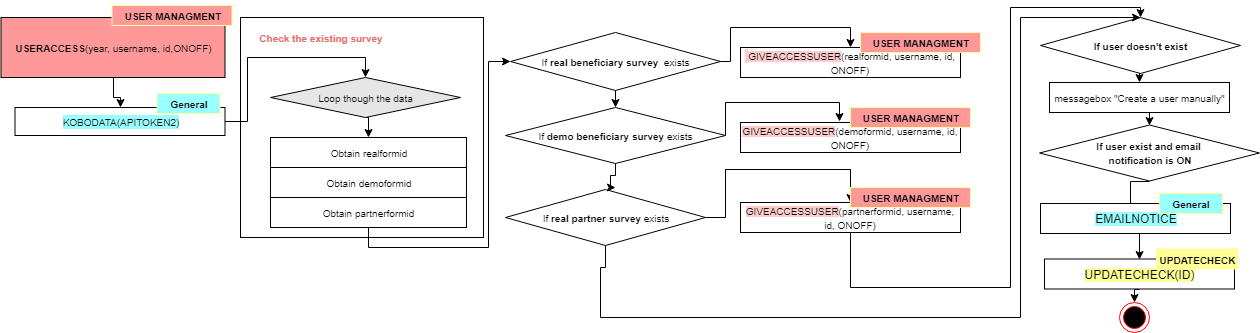
The block diagram of addUserAccess function¶
Warning
Update the function names
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | function addUserAccess(year, username, id, ONOFF)
{
/*Checking the existing beneficiary surveys to add users*/
var jsonData = KOBOFORM(key["APITOKEN2"]);
var r = 0,
rr = 0,
pr = 0;
var realformid,
demoformid,
partnerformid;
for (i = 0; i < jsonData.length; i++) {
if (jsonData[i]["title"].indexOf(year) > -1 && jsonData[i]["title"].indexOf("TRAINING (DEMO)") == -1 && jsonData[i]["title"].indexOf("Beneficiary") > -1) {
r = 1;
realformid = jsonData[i]["formid"];
}
if (jsonData[i]["title"].indexOf(year) > -1 && jsonData[i]["title"].indexOf("TRAINING (DEMO)") > -1) {
rr = 1;
demoformid = jsonData[i]["formid"];
}
if (jsonData[i]["title"].indexOf(year) > -1 && jsonData[i]["title"].indexOf("Partner") > -1) {
pr = 1;
partnerformid = jsonData[i]["formid"];
}
}
/*IF SURVEY IS CONFIRMED, ADD USERS*/
if (r == 1) {
result = GIVEACCESSUSER(realformid, username, id, ONOFF);
}
if (rr == 1) {
result = GIVEACCESSUSER(demoformid, username, id, ONOFF);
}
if (pr == 1) {
result = GIVEACCESSUSER(partnerformid, username, id, ONOFF);
}
if (result == 2 && ONOFF == "ON") { Browser.msgBox("Username does not exist. First, create username manually in KoboToolbox."); }
if (result == 1 && ONOFF == "ON" && key["EMAIL"] == "ON") {
generateEmailNotice(id,
"User Access Granted in Kobo",
"Username: " + username + " has been authorised to access Customised Surveys in Kobo",
"0",
"Thank you very much for submitting the " + year + " " + key["SECTOR"] + " Monitoring Template." +
"<br><br>We confirm the receipt and please find the PDF version for your kind attention." +
"<br><br>Please kindly let us know in case you find any information missing, or parts not reflecting correctly what you submitted." +
"<br><br>Based on the information submitted in this template, your customized surveys are developed. Please kindly find your ID and password to access your Customised Beneficiary and Partner Surveys." +
"<br><br><b>Username</b>: " + username + " (<i>Note: all in lowercase</i>)" +
"<br><b>Password</b>: 1951" +
"<br>Please keep these credentials confidential." +
"<br><br>To access your surveys, please install Apps called “KoBoCollect” in your smartphone/tablets (<i>Note: Android only</i>) and download your customized survey, by executing the following 2 tasks explained in the Kobo User guidance." +
"<br><br><b>Step.1</b>: Installing KoboCollect in your mobile" +
"<br><b>Step.2</b>: Setting up your customised beneficiary / partner survey" +
"<br> <b>2a</b>. Training survey (For Training Only)" +
"<br><br>Should you encounter any technical challenges, please do not hesitate to contact us." +
"<br><br>We would like to organize a brief training session on how to use Kobo and the customised surveys through a skype or Webex call in the coming weeks. Please let us come back to you with more details on this." +
"<br><br>Thank you and best regards,");
}
updateOnCustomisation(id);
if (result == 1) { updateLog("User access updated: " + year + " " + username + " " + ONOFF); }
return result;
}
|
Function addUserAccessCompletedTemplate¶
-
addUserAccessCompletedTemplate
(formid, username, id, ONOFF)¶ The function coordinates adding users to completed template
- Arguments
formid (type) – id of template
username (object) – current username
id (type) – id of data
ONOFF (type) – access with status ON/OFF (DataEntry/Readonly)
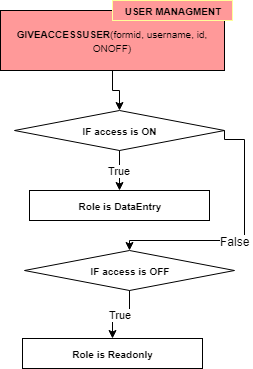
The block diagram¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | function addUserAccessCompletedTemplate(formid, username, id, ONOFF) {
var kkk = 'UNA' + id;
var url = 'https://kobocat.unhcr.org/api/v1/forms/' + formid + '/share';
if (ONOFF == "ON") {
var requestBody = {
"username": username,
"role": "dataentry"
};
}
if (ONOFF == "OFF") {
var requestBody = {
"username": username,
"role": "readonly"
};
}
var option = {
"method": "POST",
"payload": requestBody,
"headers": {
"Authorization": "Token " + key["APITOKEN2"]
}
};
try {
var json = UrlFetchApp.fetch(url, option);
}
catch (e) {
keyinputs[kkk] = "NO";
key[kkk] = "NO";
return 2;
}
return 1;
}
|
Function updateUsername¶
-
updateUsername
(pkey, skip)¶ The function checks if there is any change of username in the Control Panel
- Arguments
pkey (Object) – the list of key properties and their values
skip (string) – staus of manual update
- Returns
keyinputs – list of key parameters
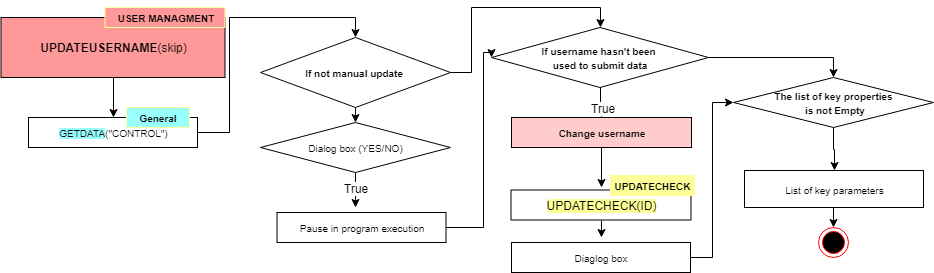
The block diagram of updateUsername function¶
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 | function updateUsername(skip, pkey) {
if (pkey != null) { key = pkey };
/*For manual click*/
if (skip != "skip") {
var r = Browser.msgBox("Warning: You can change username only once at a time", "You will not be allowed to change username again until all the recustomisation process finishes. Are you sure to proceed?", Browser.Buttons.YES_NO);
if (r == "no") { return; }
SpreadsheetApp.flush();
Utilities.sleep(2000);
}
/*For manual update: If customise has not been yet done for the first time and there us not previous username, so this part will be skipped.*/
var data = getData("CONTROL");
{ /*USERNAME CHANGE YN*/
for (di = 2; di < data.length; di++) {
if (data[di][data[0].indexOf("PROGRAMME_SYNOPSIS/PROGRAMME_INFORMATION/Year")] == "2017" || parseInt(data[di][data[0].indexOf("PROGRAMME_SYNOPSIS/PROGRAMME_INFORMATION/Year")]) < parseInt(key["AUTOYEAR"])) { continue; }
var kk = 'UN' + data[di][data[0].indexOf("_id")];
var kko = 'UNO' + data[di][data[0].indexOf("_id")];
var k = 'TEMP' + String(data[di][data[0].indexOf("_id")]);
var kkk = 'UNA' + String(data[di][data[0].indexOf("_id")]);
if (key[kk] != null && key[kk] != data[di][data[0].indexOf("Username")]) {
if (key["UNA" + data[di][data[0].indexOf("_id")]] == "LOCKED") {
Browser.msgBox("Warning [" + key[kk] + "]: The username has been already used to submit the data on surveys and should not be changed. The request will be canceled.");
var control = ss.getSheetByName("CONTROL");
control.getRange(di + 1, data[0].indexOf("Username") + 2).setValue(key[kk]);
}
else {
var old = key[kko];
if (key[kkk] != "Changing") { keyinputs[kko] = key[kk]; key[kko] = key[kk]; };
keyinputs[kk] = data[di][data[0].indexOf("Username")];
key[kk] = data[di][data[0].indexOf("Username")];
if (yearcheck(data[di][data[0].indexOf("PROGRAMME_SYNOPSIS/PROGRAMME_INFORMATION/Year")]) == 1) { keyinputs[kkk] = "Changing"; key[kkk] = "Changing"; }
if (yearcheck(data[di][data[0].indexOf("PROGRAMME_SYNOPSIS/PROGRAMME_INFORMATION/Year")]) == 1) {
var id = data[di][data[0].indexOf("_id")];
updateLog("Username changed: " + old + " to " + data[di][data[0].indexOf("Username")]);
updateOnCustomisation(id);
var htmlOutput = HtmlService
.createHtmlOutput("<style>p { margin-left: 0pt; margin-top: 5pt;margin-bottom: 5pt;color: black; font: 16px bold, Arial; }</style><p>Completed [" + key[kk] + "→" + data[di][data[0].indexOf("Username")] + "] : Username will be changed. The survey will be recustomised in the next task update.<br><br>Please <b>delete the old user access</b> if necessary (if the old username was given access already) and add the new user access manually through <a href='https://kobo.unhcr.org/' target='_blank'>KoboToolbox</a> for each of the 3 surveys.</p>")
.setWidth(500)
.setHeight(120);
SpreadsheetApp.getUi().showModalDialog(htmlOutput, 'Username changed');
}
}
}
/*ENDLINE Databridge passing ON/OFF*/
{
var eof = "EOF" + String(data[di][data[0].indexOf("_id")]);
if (key[eof] != null && key[eof] != data[di][data[0].indexOf("EOF")].toString().toLowerCase()){
keyinputs["LASTDATA" + String(data[di][data[0].indexOf("_id")])] = 0;
}
key[eof] = data[di][data[0].indexOf("EOF")];
keyinputs[eof] = key[eof];
}
}
}
if (pkey != null) { return (keyinputs); }
}
|